Goal: To be able display numbers and text onto a screen
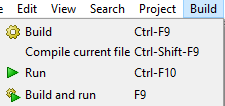
In order for a computer to take a file and execute its code, it must be told to build and run the code. On Code Blocks and Visual Studio, press F9 or click the Build and run option on the Build drop-down menu to build and run a code file.
When told to build and run a file, the computer will create an .exe file located alongside the .cpp file. However, .exe files cannot be saved onto a school computer. Thus, the .cpp file should be located on an external hard drive such as a USB when using school computers.
Running the basic skeleton listed previously will create a window resembling the following:
This window is known as the console. This is the text-based interface that accepts inputs from users and displays output values onto the screen. (Graphics will be done at a later time.) The text from the console shown above only appears once the computer finishes running the file.
-
Process returned 0 (0x0)
corresponds to thereturn 0;
line in the main function of the code file. -
The execution time is the amount of time the computer took to run the program.
-
Pressing any key will close the console window.
Outputting Values
The simplest command for C++ that outputs text onto the console is the
cout
command. cout
is a command that is not located in the Standard
Library. Instead, it is located in the <iostream>
library.
In order to add any library into a C++ file, add
#include
followed by the name of the library, at the top of the
file.
<span id="L1" class="line"><span class="cp">#include <iostream></span></span>
Note
|
Along with using namespace std; all #include statements should be
placed at the beginning of the file
|
cout
stands for “Console OUTput”, and it allows the console
to output text and numbers onto the screen. In code, the cout
command is
formatted like so:
<span id="L1" class="line"><span class="n">cout</span> <span class="o"><<</span> <span class="n">Output</span> <span class="o"><<</span> <span class="n">endl</span><span class="p">;</span></span>
The output is enclosed between two <<
and can be one of 2 types.
-
Computers could output numbers (real or integer) onto the screen
-
Text (also known as string literals) can be outputted onto the screen
-
String literals must be enclosed by quotation marks (
""
)
-
|
This marks the beginning of the output command and tells the console that a value will be outputted |
|
These symbols must be placed in between all outputs in the command. Tip: to remember the direction of the symbol, when looking from left to right, the symbol goes outwards |
|
This tells the console to skip to the next line. This part is not required in the syntax of cout. |
Note
|
Like all other commands on C++, the cout command must end with
a semicolon (; ). This marks the end of a command for a compiler.
|
<span id="L1" class="line"><span class="cp">#include <iostream> // library with cout</span></span>
<span id="L2" class="line"><span class="k">using</span> <span class="k">namespace</span> <span class="n">std</span><span class="p">;</span></span>
<span id="L3" class="line"></span>
<span id="L4" class="line"><span class="kt">int</span> <span class="nf">main</span><span class="p">()</span> <span class="p">{</span></span>
<span id="L5" class="line"> <span class="n">cout</span> <span class="o"><<</span> <span class="s">"Line 1"</span> <span class="o"><<</span> <span class="n">endl</span><span class="p">;</span> <span class="c1">// Text on line 1</span></span>
<span id="L6" class="line"> <span class="n">cout</span> <span class="o"><<</span> <span class="mi">12</span><span class="p">;</span> <span class="c1">// There is no endl</span></span>
<span id="L7" class="line"> <span class="n">cout</span> <span class="o"><<</span> <span class="mf">3.45</span><span class="p">;</span> <span class="c1">// outputs on 2nd line</span></span>
<span id="L8" class="line"> <span class="k">return</span> <span class="mi">0</span><span class="p">;</span> <span class="c1">// ends program</span></span>
<span id="L9" class="line"><span class="p">}</span></span>
Line 1 123.45
Multiple outputs can be displayed using one command simply be separating
each output with <<
. The following code has an identical output as the
code above.
<span id="L1" class="line"><span class="cp">#include <iostream></span></span>
<span id="L2" class="line"><span class="k">using</span> <span class="k">namespace</span> <span class="n">std</span><span class="p">;</span></span>
<span id="L3" class="line"></span>
<span id="L4" class="line"><span class="kt">int</span> <span class="nf">main</span><span class="p">()</span> <span class="p">{</span></span>
<span id="L5" class="line"> <span class="n">cout</span> <span class="o"><<</span> <span class="s">"Line 1"</span> <span class="o"><<</span> <span class="n">endl</span> <span class="o"><<</span> <span class="mi">12</span> <span class="o"><<</span> <span class="mf">3.45</span><span class="p">;</span></span>
<span id="L6" class="line"> <span class="k">return</span> <span class="mi">0</span><span class="p">;</span></span>
<span id="L7" class="line"><span class="p">}</span></span>
In Summary:
-
Building and running a code file will create a .exe file and open a window called a console
-
The console is able to accept user inputs and display text and values
-
The
cout
command tells the computer to output a value onto the console window -
Putting
#include <iostream>
in the file will addcout
to the computer’s list of known commands -
Text outputs must be enclosed by quotation marks
-
All commands must end with a semicolon
Practice Questions
-
Create a C++ file called HelloWorld.cpp that outputs "Hello, World!" onto the screen
-
Explain the difference between the two statements and their outputs.
-
cout << "08" << endl;
-
cout << 08 << endl;
-
-
Predict what the console will output given the following code:
<span id="L1" class="line"><span class="cp">#include <iostream></span></span> <span id="L2" class="line"><span class="k">using</span> <span class="k">namespace</span> <span class="n">std</span><span class="p">;</span></span> <span id="L3" class="line"></span> <span id="L4" class="line"><span class="kt">int</span> <span class="nf">main</span><span class="p">()</span> <span class="p">{</span></span> <span id="L5" class="line"> <span class="n">cout</span> <span class="o"><<</span> <span class="s">"01"</span> <span class="o"><<</span> <span class="n">endl</span> <span class="o"><<</span> <span class="mi">12</span><span class="p">;</span></span> <span id="L6" class="line"> <span class="n">cout</span> <span class="o"><<</span> <span class="s">" "</span> <span class="o"><<</span> <span class="mf">3.58</span><span class="p">;</span></span> <span id="L7" class="line"> <span class="n">cout</span> <span class="o"><<</span> <span class="n">endl</span> <span class="o"><<</span> <span class="n">endl</span> <span class="o"><<</span> <span class="s">"13"</span><span class="p">;</span></span> <span id="L8" class="line"> <span class="k">return</span> <span class="mi">0</span><span class="p">;</span></span> <span id="L9" class="line"><span class="p">}</span></span>